Comment form in WordPress is… adequate. It does its job but leaves a lot of room for improvement. And our first improvement is implementing better formatting like Markdown.
Markdown is a syntax for simple formatting. For example **this text**
becomes bold and *this one*
becomes italic.
Let’s implement it:
Step 1: Check if your theme uses comment_text()
Open comments.php
in your theme and check how does it outputs the comment content. Does it uses comment_text()
?
Yes? Proceed to next step.
No? Then you need to wrap the code like shown below:
...
// If original code is like this:
echo $comment->comment_content
// Wrap it like this:
echo apply_filters( 'comment_text', $comment->comment_content );
Step 2: Add Markdown parser
Hook into comment_text
and apply the Markdown while removing the other filters.
add_action( 'wp', 'comment_md_init', 9999 );
function comment_md_init() {
global $post;
// abort if in blog page or no comment yet
if( is_home() || $post->comment_count <= 0 ) { return; }
require_once 'parsedown.php'; // download the file below
// Remove escaping some symbols
remove_filter( 'comment_text', 'wptexturize', 10 );
// Remove auto anchor link
remove_filter( 'comment_text', 'make_clickable', 9 );
// Remove auto <p> tag
remove_filter( 'comment_text', 'wpautop', 30 );
// Markdown formatting
add_filter( 'comment_text', 'comment_md_format' );
}
// Format the markdown
function comment_md_format( $text ) {
$pd = new \Parsedown();
return $pd->text( $text );
}
Step 3: Try it Out
Here’s a Markdown Cheatsheet. Go try some in your comment form.
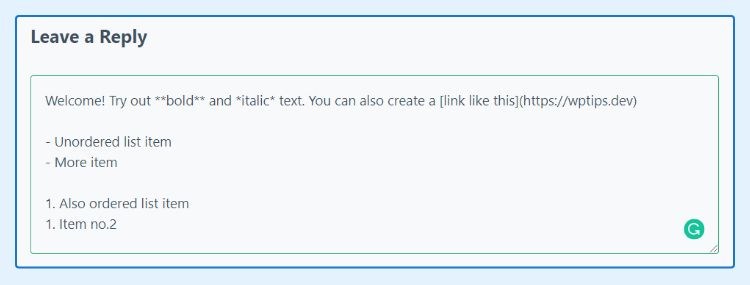
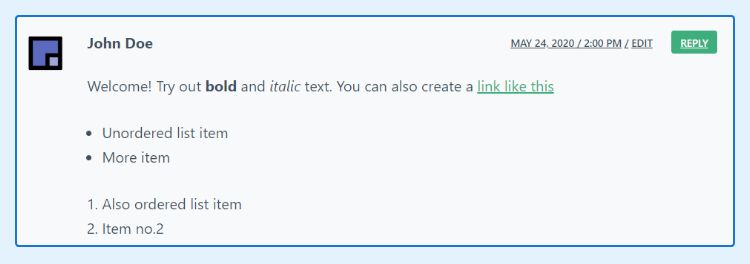
Done! You might need to change the CSS to make it looks better.
Doesn’t Work or Formatting Messy?
Check if there’s another filter that overrides your parser. At the bottom of your comment_md_init
function, add this snippet:
// at the bottom of comment_md_init() function
global $wp_filter;
var_dump( $wp_filter['comment_text'] );
Refresh your page and see the order of filters. Remove the one that you think is the problem and try again.
Step 4 (Optional): Let the visitors know that it supports Markdown
Below are two recommended ways to do it, pick one:
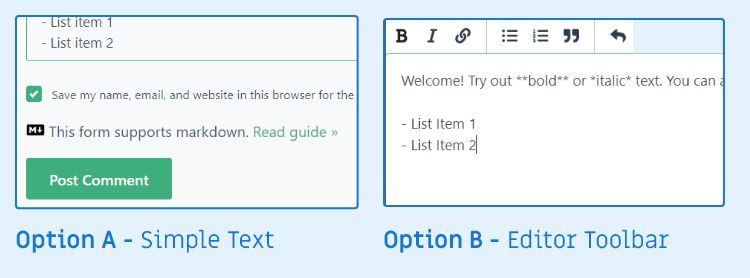
OPTION A – Simple Text
This is as simple as it gets. Feel free to change the text.
add_filter( 'comment_form_defaults', 'comment_md_add_guide' );
function comment_md_add_guide( $defaults ) {
$icon = '<svg width="20px" height="15px" xmlns="http://www.w3.org/2000/svg" viewBox="0 0 640 512">
<path d="M593.8 59.1H46.2C20.7 59.1 0 79.8 0 105.2v301.5c0 25.5 20.7 46.2 46.2 46.2h547.7c25.5 0 46.2-20.7 46.1-46.1V105.2c0-25.4-20.7-46.1-46.2-46.1zM338.5 360.6H277v-120l-61.5 76.9-61.5-76.9v120H92.3V151.4h61.5l61.5 76.9 61.5-76.9h61.5v209.2zm135.3 3.1L381.5 256H443V151.4h61.5V256H566z"/>
</svg>';
$text = 'This form supports markdown. <a href="https://commonmark.org/help/" target"_blank">Read guide ยป</a>';
$guide = "<p class='comment-md-guide'> $icon <span> $text </span> </p>";
$defaults['comment_notes_after'] .= $guide;
return $defaults;
}
OPTION B – Editor Toolbar
The snippet below will apply hEditor to the comment form. It’s a very lightweight script and the same one used by our comment form.
add_action( 'wp_enqueue_scripts', 'comment_md_enqueue_toolbar', 9999 );
function comment_md_enqueue_toolbar() {
global $post;
// If comment is open
if( isset( $post->comment_status ) && $post->comment_status == 'open' ) {
wp_enqueue_style( 'h-editor', 'https://hrsetyono.github.io/cdn/h-editor.css', [] );
wp_enqueue_script( 'h-editor', 'https://hrsetyono.github.io/cdn/h-editor-wp.js', [], null, true );
wp_localize_script( 'h-editor', 'localizeEditor', [
'textareaSelector' => '#comment',
'buttons' => [ 'bold', 'italic', 'link', '|', 'bullist', 'numlist', 'quote', '|', 'undo' ],
// Other buttons:
// h1, h2, h3, image, code, pre, hr, strike, redo
] );
}
}
Conclusion
I hope Markdown can be natively supported for Comment. But for now, we can use this tutorial which doesn’t leave any noticeable footprint. Especially if you pick Option A in Step 4.
Also do not be afraid of implementing this on a non-techie website. Even if they don’t use any Markdown, it still functions like the old comment form.
Let me know if you have trouble or suggestion in the comment below ๐
As I has read all the code but I have one problem I don’t have WordPress Programming knowledge so, can you please suggest me the Plugin or share the post if you have any already posted. Thank you.
Hi Shazaib, Unfortunately there's no plugin for the above code.
If you have knowledge in other programming language, WP isn't that hard. It's still PHP at the end of day
Well done ๐ I notice that your website also implements Markdown on comments ๐ This should be one of those mandatory things these days, Markdown is here to stay, and, sincerely, no other markup language has caught on, even though one might argue that Markdown is not the best solution out there (mostly because there are so many dialects of it!)
But it certainly beats having to type just a single asterisk instead of plain HTML
<em>
or BBCode's[i]
โ two of the most popular markups you find on comments/post articles these days.With the advent of things like Reddit or Discourse (or even Slack, Discord, and Keybase!) and even some minimalist support for Markdown in WhatsApp itself, I think that the Age of Markdown has finally arrived and is here to stay.
Now if we could only persuade MediaWiki to do the same... lol
Kudos to
hEditor
, btw. I hadn't come across it before. I appreciate how lightweight it is and how well-suited it is to the task โ and, of course, an alternative to have the full-blown TinyMCE editor on the comments section as well...Granted, I miss a "preview" function, but that's true of so many other WP comment systems, when only after submitting you have an idea on what you've submitted... and then it's too late to edit it!
Thanks for the praise! Yes, markdown is really neat and more widely used now.
I was looking for a lightweight markdown editor and can't find one that best suit me. So I have to fork one and made hEditor.
A preview would be nice, but that would make the library not lightweight anymore